In this tutorial, we'll talk about How to Upload Image to Firebase Storage and save URL to Firestore in React.js?. The users to upload images, files and data will be stored on Firebase Cloud Storage bucket. Scroll down to the page so you can see the demo and full source code available for this.
Install and Create React App
First, we’ll create a new React application using the following command in the terminal window:
npx create-react-app foldername
cd foldername
Install Firebase package in React
npm install --save firebase
npm start
This command run our project, a new browser tab will automatically open on our computer's default browser that points to "http://localhost:3000".
Next, Project structure your folder as follows.
src
├── App.css
├── App.js
├── firebase-config.js
├── index.css
└── index.js
Now go to your firebase console and open the storage page in the firebase project and create the new folder name (uploads). Enter it. After you create an uploads folder name, then go to change your storage security rules, read and write, allow both as true. After updating the code, click on publish.
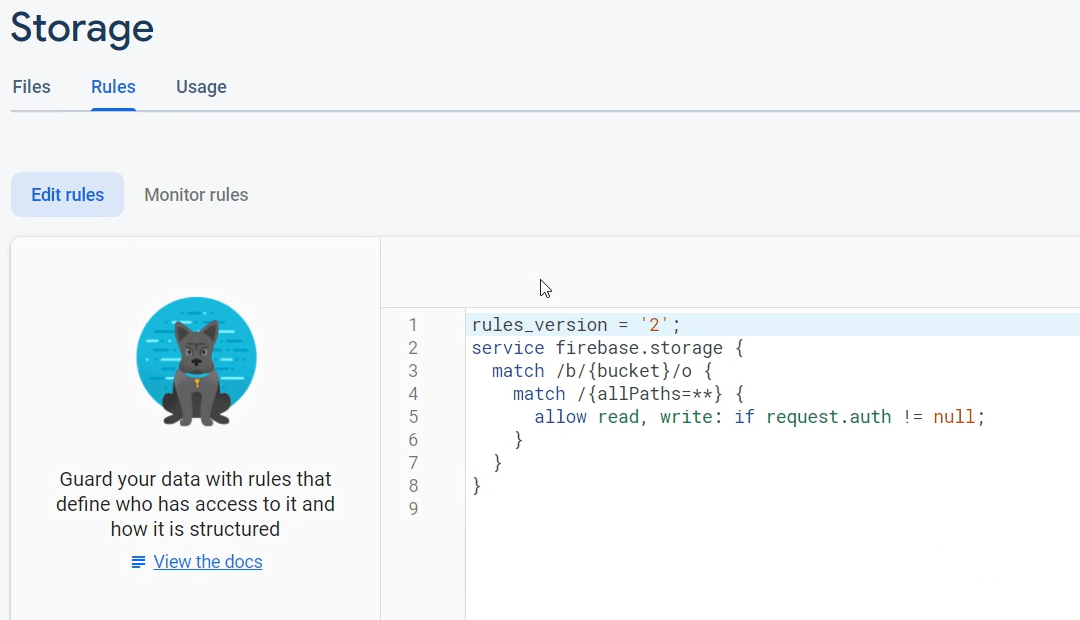
Change firebase storage security rules to:
rules_version = '2';
service firebase.storage {
match /b/{bucket}/o {
match /{allPaths=**} {
allow read, write: if true;
}
}
}
Next, Open src/firebase-config.js. Update this file with the following code.
firebase-config.jsimport firebase from 'firebase/compat/app'; import 'firebase/compat/firestore'; const firebaseApp = firebase.initializeApp({ apiKey: "AIzaSyA9KbtwienZo4Mw2bBgK0NOJgylyHcUqvw", authDomain: "react-test-a85c3.firebaseapp.com", projectId: "react-test-a85c3", storageBucket: "react-test-a85c3.appspot.com", messagingSenderId: "569657970297", appId: "1:569657970297:web:3b589cf13de06aeedc0ea8" }); const db = firebaseApp.firestore(); export default db;
Next, Open src/App.js . Update this file with the following code.
App.jsimport React, { useState, useEffect } from 'react'; import db from './firebase-config'; import firebase from 'firebase/compat/app'; import { getStorage, ref, uploadBytesResumable, getDownloadURL } from "firebase/storage"; import './App.css'; const App = () => { const [userInfo, setuserInfo] = useState({ title: '', }); const onChangeValue = (e) => { setuserInfo({ ...userInfo, [e.target.name]:e.target.value }); } const [isUsers, setUsers] = useState([]); {/* Fetch ------------------------------------------- */} useEffect(() => { db.collection('users').orderBy('datetime', 'desc').onSnapshot(snapshot => { setUsers(snapshot.docs.map(doc => { return { id: doc.id, title: doc.data().title, image: doc.data().images, datetime: doc.data().datetime } })) }) }, []); //---------------------------------------------------------- const [isfile, setFile] = useState(null); const handleImageAsFile = (e) => { setFile(e.target.files[0]); } {/* Insert ------------------------------------------- */} const addlist = async(event) => { try { event.preventDefault(); let file = isfile; const storage = getStorage(); var storagePath = 'uploads/' + file.name; const storageRef = ref(storage, storagePath); const uploadTask = uploadBytesResumable(storageRef, file); uploadTask.on('state_changed', (snapshot) => { // progrss function .... const progress = (snapshot.bytesTransferred / snapshot.totalBytes) * 100; console.log('Upload is ' + progress + '% done'); }, (error) => { // error function .... console.log(error); }, () => { // complete function .... getDownloadURL(uploadTask.snapshot.ref).then((downloadURL) => { console.log('File available at', downloadURL); db.collection('users').add({ title: userInfo.title, images: downloadURL, datetime: firebase.firestore.FieldValue.serverTimestamp() }) setuserInfo({ ...userInfo, title:'', }); setFile(null); }); }); } catch (error) { throw error;} } return (<> <div className="App"> <h1> React Firebase storage Image Upload </h1> <div className="wrapper"> {/* Insert users -------------------------------------------*/} <form onSubmit={addlist}> <input type="text" id="title" name="title" value={userInfo.title} onChange={onChangeValue} placeholder=" Title " required /> <input type="file" accept=".png, .jpg, .jpeg" onChange={handleImageAsFile}/> <button type="submit" className="btn__default btn__add" > Upload </button> </form> </div> {/* Fetch users ------------------------------------------------*/} {isUsers.map((items,index) => ( <div key={items.id} > <div className="wrapper__list"> <p><b> Title : </b> {items.title}</p> <img src={items.image} alt=""/> </div> </div> ))} </div> </>) } export default App
Related keywords
- How to do image upload with firebase in react
- How to upload files in firebase storage using ReactJS
- react-firebase-file-uploader
- Upload files with Cloud Storage on Web - Firebase
- Introduction to Firebase Storage #1: Uploading Files
- How to add image in Firebase database
- Android: How to Upload an image on Firebase storage?
- How to store and retrieve images from Firebase Database
- Firebase upload image and get URL
- Learn Firebase Cloud Storage Quickly