In this post, we are going to see How to use Firebase Firestore Databse CRUD (Create Read Update and Delete) Operations with ReactJS. It is developed by Google for creating web and mobile (IOS & Android) applications. React Firestore is very simple. Just, show the list of data, add, edit, delete and show the data details. Scroll down to the page so you can see the full source code available for this.
Install and Create React App
Step 1: First, we’ll create a new React application using the following command in the terminal window:
npx create-react-app foldername
Step 2: Next, go to the newly created app folder.
cd foldername
Step 3: Now, run the React application using the following command in the terminal window from the root directory of the project.
npm start
This command run our project, a new browser tab will automatically open on our computer's default browser that points to "http://localhost:3000".
Firebase Setup
How to set up a React App to Firebase hosting for free
Install Firebase package in React
first of all install Firebase npm package using the following command in the terminal window:
npm install --save firebase
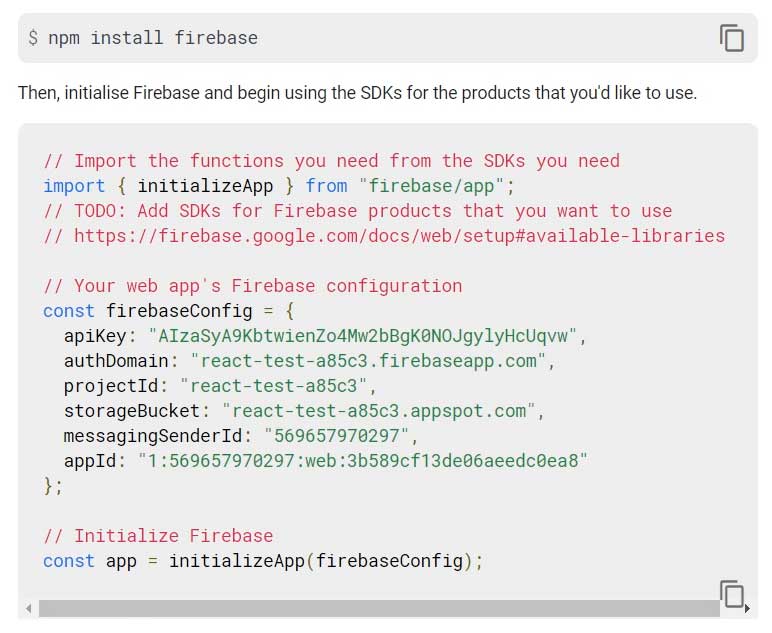
Next, Project structure your folder as follows.
src
├── App.css
├── App.js
├── firebase-config.js
├── index.css
└── index.js
Next, Open src/firebase-config.js. Update this file with the following code.
firebase-config.jsimport firebase from 'firebase/compat/app'; import 'firebase/compat/firestore'; const firebaseApp = firebase.initializeApp({ apiKey: "AIzaSyA9KbtwienZo4Mw2bBgK0NOJgylyHcUqvw", authDomain: "react-test-a85c3.firebaseapp.com", projectId: "react-test-a85c3", storageBucket: "react-test-a85c3.appspot.com", messagingSenderId: "569657970297", appId: "1:569657970297:web:3b589cf13de06aeedc0ea8" }); const db = firebaseApp.firestore(); export default db;
App.jsimport React, { useState, useEffect } from 'react'; import db from './firebase-config' import firebase from 'firebase/compat/app'; import './App.css'; const App = () => { const [userInfo, setuserInfo] = useState({ title: '', description: '', }); const [isUsers, setUsers] = useState([]); const [isOpen, setOpen] = useState(false); const [isEdit, setEdit] = useState(null); const onChangeValue = (e) => { setuserInfo({ ...userInfo, [e.target.name]:e.target.value }); } {/* Fetch ------------------------------------------- */} useEffect(() => { db.collection('users').orderBy('datetime', 'desc').onSnapshot(snapshot => { setUsers(snapshot.docs.map(doc => { return { id: doc.id, title: doc.data().title, description: doc.data().description, datetime: doc.data().datetime } })) }) }, []); {/* Insert ------------------------------------------- */} const addlist = async(event) => { try { event.preventDefault(); await db.collection('users').add({ title: userInfo.title, description: userInfo.description, datetime: firebase.firestore.FieldValue.serverTimestamp() }) setuserInfo({...userInfo, title:'', description: '', }); } catch (error) { throw error;} } {/* Edit ------------------------------------------- */} const Update = (items) => { setOpen(true); setEdit(items.id); setuserInfo({...userInfo, title:items.title, description: items.description, }); } const editlist = async(event) => { try { event.preventDefault(); db.collection('users').doc(isEdit).update({ title: userInfo.title, description: userInfo.description, }); setOpen(false); setEdit(null); setuserInfo({...userInfo, title:'', description: '', }); } catch (error) { throw error;} } {/* Delete ------------------------------------------- */} const Delete = (id) => { db.collection('users').doc(id).delete().then(res => { console.log('Deleted!', res); }); } return (<> <div className="App"> <h1> React Firebase Firestore CRUD Operations </h1> <div className="wrapper"> {/* Insert users -------------------------------------------*/} {isOpen === false && <form onSubmit={addlist}> <input type="text" id="title" name="title" value={userInfo.title} onChange={onChangeValue} placeholder=" Title " required /> <textarea id="desc" name="description" value={userInfo.description} onChange={onChangeValue} placeholder=" Description " /> <button type="submit" className="btn__default btn__add" > Add </button> </form> } </div> {/* Fetch users ------------------------------------------------*/} {isUsers.map((items,index) => ( <div key={items.id} > <div className="wrapper__list"> <p><b> Title : </b> {items.title}</p> <p><b> Description : </b>{items.description}</p> <p><b> Date : </b>{items.datetime?.toDate().toLocaleDateString("en-US")}</p> <div className="update__list"> <button onClick={()=>Update(items)} className="btn__default btn__edit"> Edit </button> <button onClick={()=>Delete(items.id)} className="btn__default btn__delete"> delete </button> </div> {/* Edit users ------------------------------------------- */} {isOpen === true && isEdit === items.id && <form onSubmit={editlist}> <input type="text" id="title" name="title" value={userInfo.title} onChange={onChangeValue} placeholder=" Title " required /> <textarea id="desc" name="description" value={userInfo.description} onChange={onChangeValue} placeholder=" Description " /> <button type="submit" className="btn__default btn__add" > Save </button> </form> } </div> </div> ))} </div> </>) } export default App
Conclusion
Today we’ve Building React Firebase Firestore CRUD Web Application successfully working. Now we can Insert, update, display, delete documents and collection at ease.
Related keywords
- Firebase React CRUD tutorial
- How to use Firebase Firestore with ReactJS
- Firebase install npm package
- firestore database
- firestore database insert query
- Firestore add document with auto id
- Firestore update document by id
- Cloud Firestore — Add, Set, Update, Delete, Get data
- update cloud firestore document without id
- CRUD with Cloud Firestore using the react js
- How To Implement React CRUD Using Firebase
- React Hooks Firebase CRUD
- This website show country are united states, France, Canada, japan, New york, london, Los Angeles, Russia, Italy, Spain, Austria, Serbia, Moldova, Bosnia and Herzegovina, Albania, Lithuania, North Macedonia, Switzerland, Bulgaria, Denmark,Germany, United Kingdom.