In this post, we are going to see about implementing Stripe checkout Payment Gateway Integration in PHP. It is easily registered debit/credit card(s) with your Stripe account. It is integrate Stripe API account is used for a variety of PHP-based website including e-commerce websites, freelancers, digital marketing, and more. Scroll down to the page so you can see the full source code available for this.
Stripe is an online secure payment platform. It is easy-to-use and powerful way to accept credit cards directly on the web application worldwide. Stripe payment gateway is suitable for individuals who want to send and receive payments, sellers, business and freelancers.
Stripe Integration Steps:
Step 1 : First thing, Go to this link to create a Stripe account and login to the dashboard stripe.com
Step 2 : Get a API key from your account.
There is two Generate Stripe API Keys named secret key and publishable key.
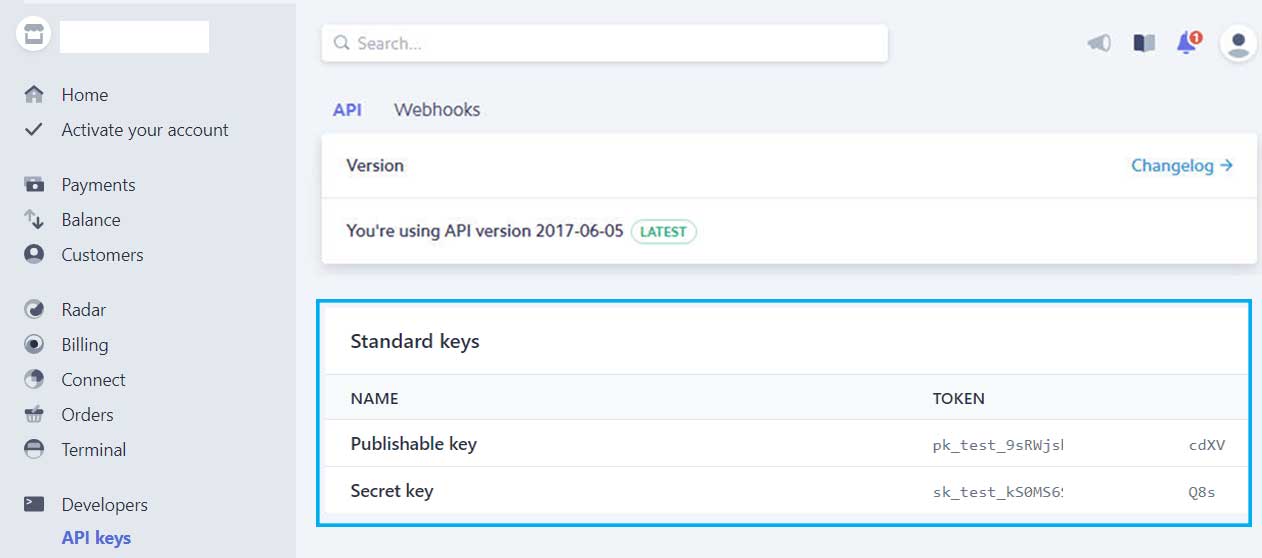
Next, Project structure your folder as follows.
stripe
├── css
└── style.css
├── stripe-php
├── cancel.php
├── config.php
├── dbConnect.php
├── index.php
├── stripe_charge.php
└── success.php
MySQL database
In the following example, we will start how to Stripe Payment Gateway Integration into the MySQL database using PHP.
- Database Name – codeat21
- Table Name – products, orders
productsCREATE TABLE `products` ( `id` int(11) NOT NULL AUTO_INCREMENT, `name` varchar(200) COLLATE utf8_unicode_ci NOT NULL, `image` varchar(255) COLLATE utf8_unicode_ci NOT NULL, `price` float(10,2) NOT NULL, `status` tinyint(1) NOT NULL DEFAULT '1' COMMENT '1=Active | 0=Inactive', PRIMARY KEY (`id`) ) ENGINE=InnoDB DEFAULT CHARSET=utf8 COLLATE=utf8_unicode_ci;
ordersCREATE TABLE `orders` ( `id` int(11) NOT NULL AUTO_INCREMENT, `name` varchar(50) COLLATE utf8_unicode_ci NOT NULL, `email` varchar(50) COLLATE utf8_unicode_ci NOT NULL, `item_number` varchar(50) COLLATE utf8_unicode_ci DEFAULT NULL, `item_name` varchar(255) COLLATE utf8_unicode_ci NOT NULL, `item_price` float(10,2) NOT NULL, `item_price_currency` varchar(10) COLLATE utf8_unicode_ci NOT NULL, `paid_amount` float(10,2) NOT NULL, `paid_amount_currency` varchar(10) COLLATE utf8_unicode_ci NOT NULL, `txn_id` varchar(100) COLLATE utf8_unicode_ci NOT NULL, `checkout_session_id` varchar(255) COLLATE utf8_unicode_ci NOT NULL, `payment_status` varchar(25) COLLATE utf8_unicode_ci NOT NULL, `created` datetime NOT NULL, `modified` datetime NOT NULL, PRIMARY KEY (`id`) ) ENGINE=InnoDB DEFAULT CHARSET=utf8 COLLATE=utf8_unicode_ci;
dbConnect.php<?php // Connect with the database $db_conn = new mysqli(DB_HOST, DB_USERNAME, DB_PASSWORD, DB_NAME); // Display error if failed to connect if ($db_conn->connect_errno) { printf("Connect failed: %s\n", $db_conn->connect_error); exit(); } ?>
config.php<?php // Product Details // Minimum amount is $0.50 US // Test Stripe API configuration define('STRIPE_API_KEY', 'Your_API_Secret_key'); define('STRIPE_PUBLISHABLE_KEY', 'Your_API_Publishable_key'); define('STRIPE_SUCCESS_URL', 'http://localhost/stripe/success.php'); define('STRIPE_CANCEL_URL', 'http://localhost/stripe/cancel.php'); // Database configuration define('DB_HOST', 'localhost'); define('DB_USERNAME', 'root'); define('DB_PASSWORD', ''); define('DB_NAME', 'codeat21'); ?>
index.php<?php // Include configuration file require_once 'config.php'; include 'dbConnect.php'; ?> <!DOCTYPE html> <html lang="en-US"> <head> <title> Stripe Checkout in PHP by codeat21.com</title> <meta charset="utf-8"> <!-- Stylesheet file --> <link href="css/style.css" rel="stylesheet"> <!-- Stripe JavaScript library --> <script src="https://js.stripe.com/v3/"></script> </head> <body class="App"> <h1>How to Integrate Stripe Payment Gateway in PHP</h1> <div class="wrapper"> <!-- Display errors returned by checkout session --> <div id="paymentResponse"></div> <?php $results = mysqli_query($db_conn,"SELECT * FROM products where status=1"); $row = mysqli_fetch_array($results,MYSQLI_ASSOC); ?> <div class="col__box"> <h5><?php echo $row['name']; ?></h5> <h6>Price: <span> $<?php echo $row['price']; ?> </span> </h6> <!-- Buy button --> <div id="buynow"> <button class="btn__default" id="payButton"> Buy Now </button> </div> </div> </div> <script> var buyBtn = document.getElementById('payButton'); var responseContainer = document.getElementById('paymentResponse'); // Create a Checkout Session with the selected product var createCheckoutSession = function (stripe) { return fetch("stripe_charge.php", { method: "POST", headers: { "Content-Type": "application/json", }, body: JSON.stringify({ checkoutSession: 1, Name:"<?php echo $row['name']; ?>", ID:"<?php echo $row['id']; ?>", Price:"<?php echo $row['price']; ?>", Currency:"<?php echo $row['currency']; ?>", }), }).then(function (result) { return result.json(); }); }; // Handle any errors returned from Checkout var handleResult = function (result) { if (result.error) { responseContainer.innerHTML = '<p>'+result.error.message+'</p>'; } buyBtn.disabled = false; buyBtn.textContent = 'Buy Now'; }; // Specify Stripe publishable key to initialize Stripe.js var stripe = Stripe('<?php echo STRIPE_PUBLISHABLE_KEY; ?>'); buyBtn.addEventListener("click", function (evt) { buyBtn.disabled = true; buyBtn.textContent = 'Please wait...'; createCheckoutSession().then(function (data) { if(data.sessionId){ stripe.redirectToCheckout({ sessionId: data.sessionId, }).then(handleResult); }else{ handleResult(data); } }); }); </script> </body> </html>
stripe_charge.php<?php // Include configuration file require_once 'config.php'; // Include Stripe PHP library require_once 'stripe-php/init.php'; // Set API key \Stripe\Stripe::setApiKey(STRIPE_API_KEY); $response = array( 'status' => 0, 'error' => array( 'message' => 'Invalid Request!' ) ); if ($_SERVER['REQUEST_METHOD'] == 'POST') { $input = file_get_contents('php://input'); $request = json_decode($input); } if (json_last_error() !== JSON_ERROR_NONE) { http_response_code(400); echo json_encode($response); exit; } $productName = $request->Name; $productID = $request->ID; $productPrice = $request->Price; $currency = $request->Currency; // Convert product price to cent $stripeAmount = round($productPrice*100, 2); if(!empty($request->checkoutSession)){ // Create new Checkout Session for the order try { $session = \Stripe\Checkout\Session::create([ 'payment_method_types' => ['card'], 'line_items' => [[ 'price_data' => [ 'product_data' => [ 'name' => $productName, 'metadata' => [ 'pro_id' => $productID ] ], 'unit_amount' => $stripeAmount, 'currency' => $currency, ], 'quantity' => 1, 'description' => $productName, ]], 'mode' => 'payment', 'success_url' => STRIPE_SUCCESS_URL.'?session_id={CHECKOUT_SESSION_ID}&getID='.$productID, 'cancel_url' => STRIPE_CANCEL_URL, ]); }catch(Exception $e) { $api_error = $e->getMessage(); } if(empty($api_error) && $session){ $response = array( 'status' => 1, 'message' => 'Checkout Session created successfully!', 'sessionId' => $session['id'] ); }else{ $response = array( 'status' => 0, 'error' => array( 'message' => 'Checkout Session creation failed! '.$api_error ) ); } } // Return response echo json_encode($response);
success.php<?php // Include configuration file require_once 'config.php'; include 'dbConnect.php'; $pageview = $_GET['getID']; $selectproduct =mysqli_query($db_conn, "select * from products where id = '$pageview' "); $rowproduct =mysqli_fetch_array($selectproduct,MYSQLI_ASSOC); $payment_id = $statusMsg = ''; $ordStatus = 'error'; // Check whether stripe checkout session is not empty if(!empty($_GET['session_id'])){ $session_id = $_GET['session_id']; // Fetch transaction data from the database if already exists $sql = "SELECT * FROM orders WHERE checkout_session_id = '".$session_id."'"; $result = $db_conn->query($sql); if ( !empty($result->num_rows) && $result->num_rows > 0) { $orderData = $result->fetch_assoc(); $paymentID = $orderData['id']; $transactionID = $orderData['txn_id']; $paidAmount = $orderData['paid_amount']; $paidCurrency = $orderData['paid_amount_currency']; $paymentStatus = $orderData['payment_status']; $ordStatus = 'success'; $statusMsg = 'Your Payment has been Successful!'; }else{ // Include Stripe PHP library require_once 'stripe-php/init.php'; // Set API key \Stripe\Stripe::setApiKey(STRIPE_API_KEY); // Fetch the Checkout Session to display the JSON result on the success page try { $checkout_session = \Stripe\Checkout\Session::retrieve($session_id); }catch(Exception $e) { $api_error = $e->getMessage(); } if(empty($api_error) && $checkout_session){ // Retrieve the details of a PaymentIntent try { $intent = \Stripe\PaymentIntent::retrieve($checkout_session->payment_intent); } catch (\Stripe\Exception\ApiErrorException $e) { $api_error = $e->getMessage(); } // Retrieves the details of customer try { // Create the PaymentIntent $customer = \Stripe\Customer::retrieve($checkout_session->customer); } catch (\Stripe\Exception\ApiErrorException $e) { $api_error = $e->getMessage(); } if(empty($api_error) && $intent){ // Check whether the charge is successful if($intent->status == 'succeeded'){ // Customer details $name = $customer->name; $email = $customer->email; // Transaction details $transactionID = $intent->id; $paidAmount = $intent->amount; $paidAmount = ($paidAmount/100); $paidCurrency = $intent->currency; $paymentStatus = $intent->status; // Insert transaction data into the database $sql = "INSERT INTO orders(name,email,item_name,item_number,item_price,item_price_currency,paid_amount,paid_amount_currency,txn_id,payment_status,checkout_session_id,created,modified) VALUES('".$name."','".$email."','".$rowproduct['name']."','".$rowproduct['id']."','".$rowproduct['price']."','".$rowproduct['currency']."','".$paidAmount."','".$paidCurrency."','".$transactionID."','".$paymentStatus."','".$session_id."',NOW(),NOW())"; $insert = $db_conn->query($sql); $paymentID = $db_conn->insert_id; $ordStatus = 'success'; $statusMsg = 'Your Payment has been Successful!'; }else{ $statusMsg = "Transaction has been failed!"; } }else{ $statusMsg = "Unable to fetch the transaction details! $api_error"; } $ordStatus = 'success'; }else{ $statusMsg = "Transaction has been failed! $api_error"; } } }else{ $statusMsg = "Invalid Request!"; } ?> <!DOCTYPE html> <html lang="en-US"> <head> <title>Stripe Payment Status - codeat21 </title> <meta charset="utf-8"> <!-- Stylesheet file --> <link href="css/style.css" rel="stylesheet"> </head> <body class="App"> <h1 class="<?php echo $ordStatus; ?>"><?php echo $statusMsg; ?></h1> <div class="wrapper"> <?php if(!empty($paymentID)){ ?> <h4>Payment Information</h4> <p><b>Reference Number:</b> <?php echo $paymentID; ?></p> <p><b>Transaction ID:</b> <?php echo $transactionID; ?></p> <p><b>Paid Amount:</b> <?php echo $paidAmount.' '.$paidCurrency; ?></p> <p><b>Payment Status:</b> <?php echo $paymentStatus; ?></p> <h4>Product Information</h4> <p><b>Name:</b> <?php echo $rowproduct['name']; ?></p> <p><b>Price:</b> <?php echo $rowproduct['price'].' '.$rowproduct['currency']; ?></p> <?php } ?> <a href="index.php" class="btn-link">Back to Product Page</a> </div> </body> </html>
cancel.php<!DOCTYPE html> <html lang="en-US"> <head> <title>Stripe Payment Status - codeat21 </title> <meta charset="utf-8"> <!-- Stylesheet file --> <link href="css/style.css" rel="stylesheet"> </head> <body class="App"> <h1>Your transaction was canceled!</h1> <div class="wrapper"> <a href="index.php" class="btn-link">Back to Product Page</a> </div> </body> </html>
Test Card Details
The following test card Details are
- CVV - any 3 digit number
- Expiry date - any month and year from the current month and year
- Visa(debit) Card 4000056655665556
- Mastercard 5555555555554444
- Mastercard (debit) 5200828282828210
- American Express 378282246310005
- Discover 6011111111111117
conclusion
In this tutorial, you learned about How to Integrate stripe checkout Payment Gateway in PHP. This payment gateway is the easiest way to accept debit card or credit card payment directly on the website application. It is add a success page and cancel page to the application we created. Change the Test API keys to Live API keys in the script.